Kate gains Support for Inline Notes
Thanks to Michal Srb and Sven Brauch for pioneering the work an a new KTextEditor interface that allows applications like Kate, KDevelop, etc. to display inline notes in a text document. As demo, we quickly prototyped one application to display colors in CSS documents:
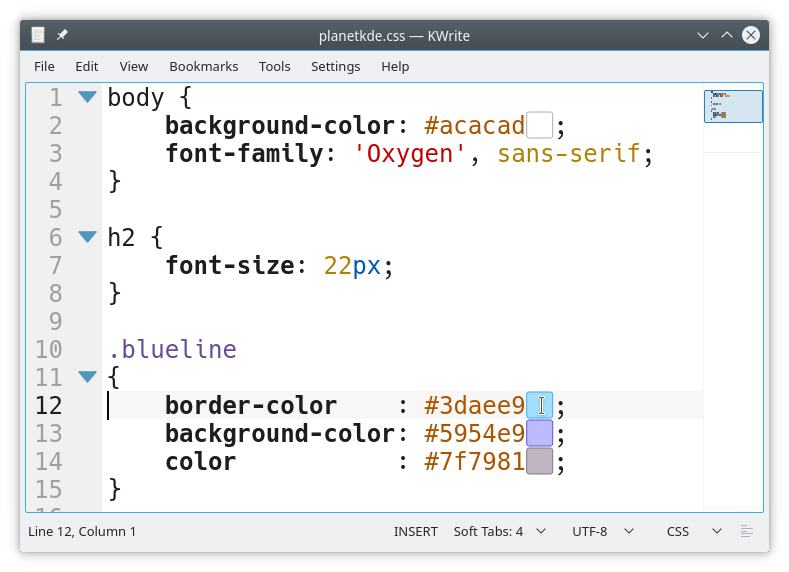
Clicking on the color rectangle will launch the color chooser:
Choosing a color and clicking OK finally adapts the color in the CSS document:
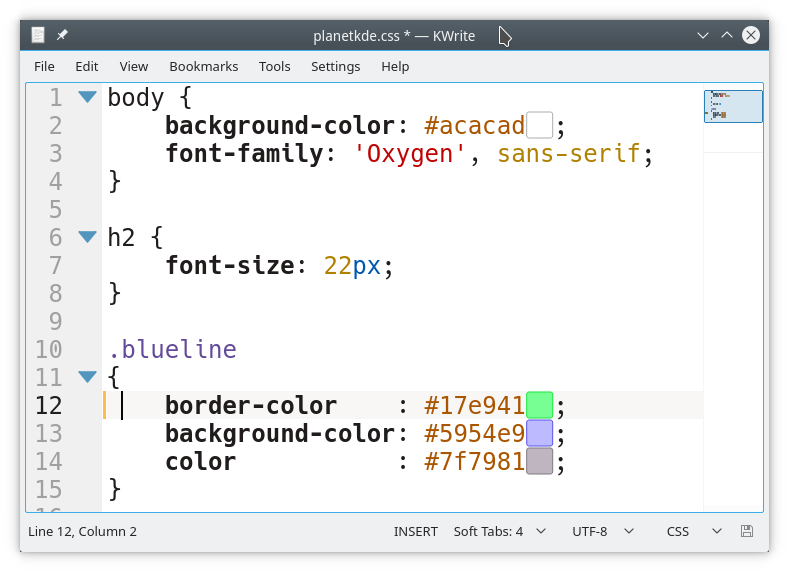
The code for this is just a demo and looks as follows:
class NoteProvider : public KTextEditor::InlineNoteProvider { public: QVector<int> inlineNotes(int line) const override { if (line == 1) return { 29 }; if (line == 11) return { 29 }; if (line == 12) return { 29 }; if (line == 13) return { 29 }; return {}; } QSize inlineNoteSize(const KTextEditor::InlineNote& note) const override { return QSize(note.lineHeight(), note.lineHeight()); } void paintInlineNote(const KTextEditor::InlineNote& note, QPainter& painter) const override { const auto line = note.position().line(); const auto color = QColor(note.view()->document()->text({line, 22, line, 29})); painter.setPen(color); painter.setBrush(color.lighter(150)); painter.drawRoundedRect(1, 1, note.width() - 2, note.lineHeight() - 2, 2, 2); } void inlineNoteActivated(const KTextEditor::InlineNote& note, Qt::MouseButtons buttons, const QPoint& globalPos) override { const int line = note.position().line(); const auto oldColor = QColor(note.view()->document()->text({line, 22, line, 29})); const auto newColor = QColorDialog::getColor(oldColor); note.view()->document()->replaceText({line, 22, line, 29}, newColor.name(QColor::HexRgb)); } void inlineNoteFocusInEvent(const KTextEditor::InlineNote& note, const QPoint& globalPos) override {} // unused in this example void inlineNoteFocusOutEvent(const KTextEditor::InlineNote& note) override {} // unused in this example void inlineNoteMouseMoveEvent(const KTextEditor::InlineNote& note, const QPoint& globalPos) override {} // unused in this example }; // later in code: auto provider = new NoteProvider(); view->registerInlineNoteProvider(provider); // final cleanup view->unregisterInlineNoteProvider(provider);
As you can see, it’s actually not much code at all: We have to derive a class from KTextEditor::InlineNoteProvider, and then register an instance of our Note Provider in the KTextEditor::View. In a next step, we implement the inlineNotes(), inlineNoteSize(), and the paintInlineNote() functions to get basic visual drawing at the desired location. The above code is just a tech-demo, since it uses hard-coded lines and color positions. Additionally, one can also track mouse events (unused in the example above). On mouse click, we open the QColorDialog to let the user choose a new color.
To give more examples of what’s possible, the initial Phabricator review requests contained many other interesting examples (the examples were really implemented). From review request D12662:
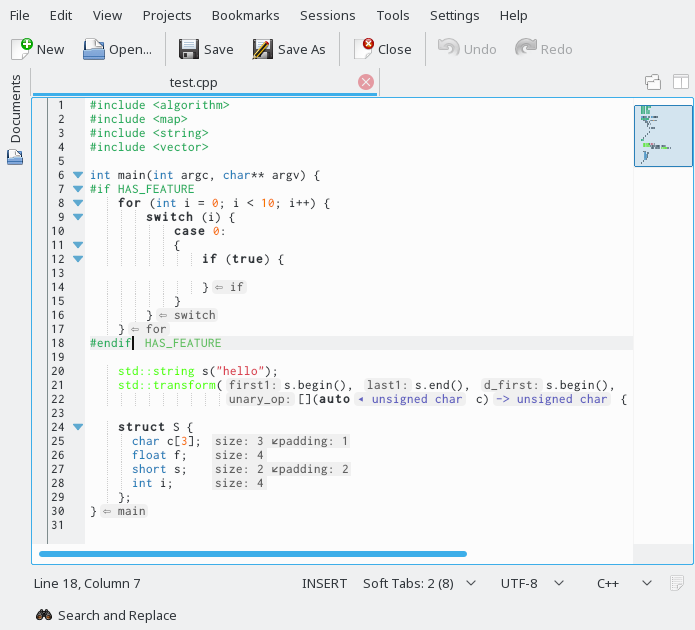
Or a KDevelop addition that adds a lot of meta information on the current code if desired:
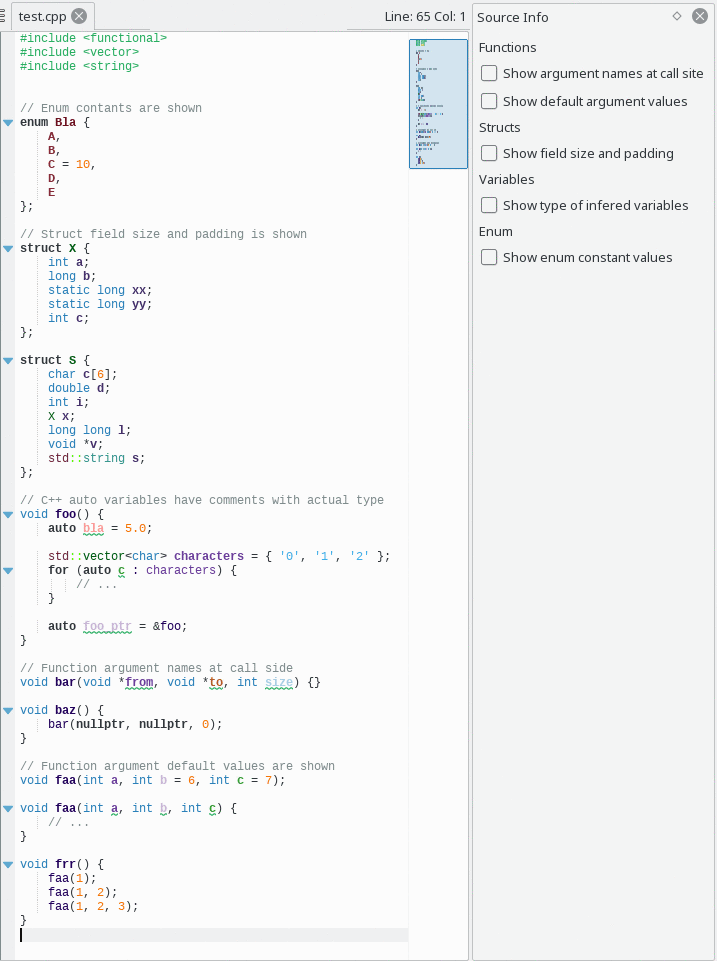
We believe this addition to the KTextEditor component has a lot of potential for nice features and plugins. Feel free to use this interfaces starting with KDE Frameworks 5.50. Happy coding! :-)
A big thanks also goes to this year’s Akademy organizers. Thanks to this event, we could meet up in person and also finalize the InlineNoteInterface, InlineNoteProvider, and InlineNote class to make it ready for public release. This again shows the importance of the yearly KDE conferences since it enables us to significantly push things forward.